摘要:
自定义环形进度条自定义圆形进度条的设计草图:12345678910111213141516171819202122232425262728293031323334363738393944041444447484950515253545556packagecom.qiao.circleprogress_forexample;importandroid.app.活动;importandroid.os
效果图:
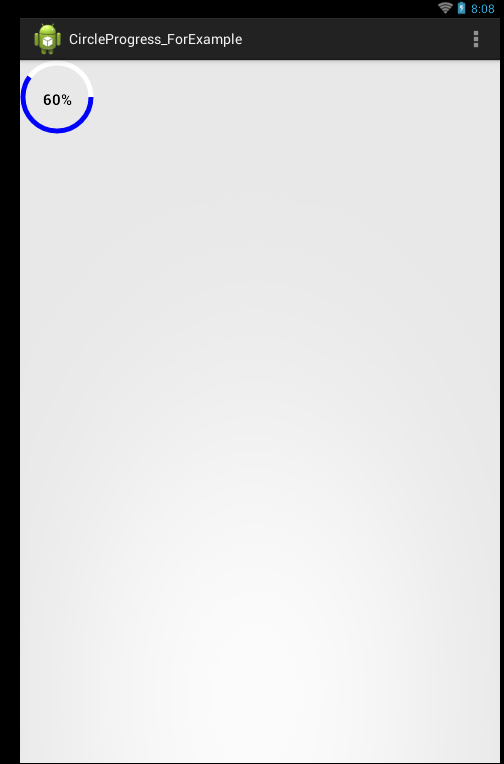
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | package com.qiao.circleprogress_forexample; import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; /** * 主页面 * @author 有点凉了 * */ public class MainActivity extends Activity { private RoundProgressBar progressBar1; private int progress = 0 ; @Override protected void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); progressBar1 = (RoundProgressBar) findViewById(R.id.progressBar1); new Thread( new Runnable() { @Override public void run() { while (progress<= 59 ) { progress+= 1 ; progressBar1.setProgress(progress); try { Thread.sleep( 100 ); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } }).start(); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true ; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true ; } return super .onOptionsItemSelected(item); } } |
自定义页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 | package com.qiao.circleprogress_forexample; import android.content.Context; import android.content.res.TypedArray; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.Paint; import android.graphics.RectF; import android.graphics.Typeface; import android.util.AttributeSet; import android.util.Log; import android.view.View; /** * 仿iphone带进度的进度条,线程安全的View,可直接在线程中更新进度 * @author 有点凉了 * */ public class RoundProgressBar extends View { /** * 画笔对象的引用 */ private Paint paint; /** * 圆环的颜色 */ private int roundColor; /** * 圆环进度的颜色 */ private int roundProgressColor; /** * 中间进度百分比的字符串的颜色 */ private int textColor; /** * 中间进度百分比的字符串的字体 */ private float textSize; /** * 圆环的宽度 */ private float roundWidth; /** * 最大进度 */ private int max; /** * 当前进度 */ private int progress; /** * 是否显示中间的进度 */ private boolean textIsDisplayable; /** * 进度的风格,实心或者空心 */ private int style; public static final int STROKE = 0 ; public static final int FILL = 1 ; public RoundProgressBar(Context context) { this (context, null ); } public RoundProgressBar(Context context, AttributeSet attrs) { this (context, attrs, 0 ); } public RoundProgressBar(Context context, AttributeSet attrs, int defStyle) { super (context, attrs, defStyle); paint = new Paint(); TypedArray mTypedArray = context.obtainStyledAttributes(attrs, R.styleable.RoundProgressBar); //获取自定义属性和默认值 roundColor = mTypedArray.getColor(R.styleable.RoundProgressBar_roundColor, Color.WHITE); roundProgressColor = mTypedArray.getColor(R.styleable.RoundProgressBar_roundProgressColor, Color.BLUE); textColor = mTypedArray.getColor(R.styleable.RoundProgressBar_textColor, Color.BLACK); textSize = mTypedArray.getDimension(R.styleable.RoundProgressBar_textSize, 15 ); roundWidth = mTypedArray.getDimension(R.styleable.RoundProgressBar_roundWidth, 5 ); max = mTypedArray.getInteger(R.styleable.RoundProgressBar_max, 100 ); textIsDisplayable = mTypedArray.getBoolean(R.styleable.RoundProgressBar_textIsDisplayable, true ); style = mTypedArray.getInt(R.styleable.RoundProgressBar_style, 0 ); mTypedArray.recycle(); } @Override protected void onDraw(Canvas canvas) { super .onDraw(canvas); /** * 画最外层的大圆环 */ int centre = getWidth()/ 2 ; //获取圆心的x坐标 int radius = ( int ) (centre - roundWidth/ 2 ); //圆环的半径 paint.setColor(roundColor); //设置圆环的颜色 paint.setStyle(Paint.Style.STROKE); //设置空心 paint.setStrokeWidth(roundWidth); //设置圆环的宽度 paint.setAntiAlias( true ); //消除锯齿 canvas.drawCircle(centre, centre, radius, paint); //画出圆环 Log.e( "log" , centre + "" ); /** * 画进度百分比 */ paint.setStrokeWidth( 0 ); paint.setColor(textColor); paint.setTextSize(textSize); paint.setTypeface(Typeface.DEFAULT_BOLD); //设置字体 int percent = ( int )((( float )progress / ( float )max) * 100 ); //中间的进度百分比,先转换成float在进行除法运算,不然都为0 float textWidth = paint.measureText(percent + "%" ); //测量字体宽度,我们需要根据字体的宽度设置在圆环中间 if (textIsDisplayable && percent != 0 && style == STROKE){ canvas.drawText(percent + "%" , centre - textWidth / 2 , centre + textSize/ 2 , paint); //画出进度百分比 } /** * 画圆弧 ,画圆环的进度 */ //设置进度是实心还是空心 paint.setStrokeWidth(roundWidth); //设置圆环的宽度 paint.setColor(roundProgressColor); //设置进度的颜色 RectF oval = new RectF(centre - radius, centre - radius, centre + radius, centre + radius); //用于定义的圆弧的形状和大小的界限 switch (style) { case STROKE:{ paint.setStyle(Paint.Style.STROKE); canvas.drawArc(oval, 0 , 360 * progress / max, false , paint); //根据进度画圆弧 break ; } case FILL:{ paint.setStyle(Paint.Style.FILL_AND_STROKE); if (progress != 0 ) canvas.drawArc(oval, 0 , 360 * progress / max, true , paint); //根据进度画圆弧 break ; } } } public synchronized int getMax() { return max; } /** * 设置进度的最大值 * @param max */ public synchronized void setMax( int max) { if (max < 0 ){ throw new IllegalArgumentException( "max not less than 0" ); } this .max = max; } /** * 获取进度.需要同步 * @return */ public synchronized int getProgress() { return progress; } /** * 设置进度,此为线程安全控件,由于考虑多线的问题,需要同步 * 刷新界面调用postInvalidate()能在非UI线程刷新 * @param progress */ public synchronized void setProgress( int progress) { if (progress < 0 ){ throw new IllegalArgumentException( "progress not less than 0" ); } if (progress > max){ progress = max; } if (progress <= max){ this .progress = progress; postInvalidate(); } } public int getCricleColor() { return roundColor; } public void setCricleColor( int cricleColor) { this .roundColor = cricleColor; } public int getCricleProgressColor() { return roundProgressColor; } public void setCricleProgressColor( int cricleProgressColor) { this .roundProgressColor = cricleProgressColor; } public int getTextColor() { return textColor; } public void setTextColor( int textColor) { this .textColor = textColor; } public float getTextSize() { return textSize; } public void setTextSize( float textSize) { this .textSize = textSize; } public float getRoundWidth() { return roundWidth; } public void setRoundWidth( float roundWidth) { this .roundWidth = roundWidth; } } |
布局:
1 2 3 4 5 6 7 8 9 10 11 12 | <LinearLayout xmlns:android= "http://schemas.android.com/apk/res/android" android:layout_width= "fill_parent" android:layout_height= "fill_parent" android:orientation= "vertical" > <com.qiao.circleprogress_forexample.RoundProgressBar android:id= "@+id/progressBar1" android:layout_width= "100dip" android:layout_height= "100dip" /> </LinearLayout> |
属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | <?xml version= "1.0" encoding= "UTF-8" ?> <resources> <declare-styleable name= "RoundProgressBar" > <attr name= "roundColor" format= "color" /> <attr name= "roundProgressColor" format= "color" /> <attr name= "roundWidth" format= "dimension" ></attr> <attr name= "textColor" format= "color" /> <attr name= "textSize" format= "dimension" /> <attr name= "max" format= "integer" ></attr> <attr name= "textIsDisplayable" format= "boolean" ></attr> <attr name= "style" > < enum name= "STROKE" value= "0" ></ enum > < enum name= "FILL" value= "1" ></ enum > </attr> </declare-styleable> </resources> |