摘要:18downvotefavorite4I'vegotafairlysimpleSpringBootwebapplication,IhaveasingleHTMLpagewithaformwithenctype="multipart/form-data".I'mgettingthiserror:Themulti-partrequestcontainedparameterdata(excludingu
| I've got a fairly simple Spring Boot web application, I have a single HTML page with a form withenctype="multipart/form-data" . I'm getting this error: The multi-part request contained parameter data (excluding uploaded files) that exceeded the limit for maxPostSize set on the associated connector.
I'm using Spring Boot's default embedded tomcat server. Apparently the defaultmaxPostSize value is 2 megabytes. Is there any way to edit this value? Doing so viaapplication.properties would be best, rather than having to create customized beans or mess with xml files. |
| | Whereever you have declared your multi-part resolver, you can change these things.– We are BorgOct 20 '15 at 11:22 | | I haven't declared it anywhere. I'm assuming that means spring boot is automatically creating and handling it. Does that mean I can't edit it?– JordanOct 20 '15 at 23:56 | | You can edit it. Find the multipart resolver and edit the value. If there is multipart support, I am sure you will find some configuration for it. You have not even posted your config in the main post, so no one can even point out what to change.– We are BorgOct 21 '15 at 7:04 |
|
| Inapplication.propertiesfile write this: # Max file size.
spring.http.multipart.max-file-size=1Mb
# Max request size.
spring.http.multipart.max-request-size=10Mb
adjust size according to your need. | | answeredOct 20 '15 at 9:36 |
|
| | Tried that, still gave me errors. I'm not actually uploading any files in the form, I just have a lot of input fields with values tens of thousands of characters long, and if I have too much text (more than 2MB of text) it crashes. Was hoping to increase the postMaxSize to more than 2MB to avoid this problem.– JordanOct 21 '15 at 0:10 | | | | The dashes should not be included in the property names. multipart.maxRequestSize=20MB multipart.maxFileSize=20MB– userM1433372Mar 25 '16 at 20:30 | | spring.http.multipart.max-file-size=1Mb # Max file size. Values can use the suffixed "MB" or "KB" to indicate a Megabyte or Kilobyte size. spring.http.multipart.max-request-size=10Mb # Max request size. Values can use the suffixed "MB" or "KB" to indicate a Megabyte or Kilobyte size. – deFreitasAug 25 '16 at 19:05 |
|
| Found a solution. Add this code to the same class running SpringApplication.run. // Set maxPostSize of embedded tomcat server to 10 megabytes (default is 2 MB, not large enough to support file uploads > 1.5 MB)
@Bean
EmbeddedServletContainerCustomizer containerCustomizer() throws Exception {
return (ConfigurableEmbeddedServletContainer container) -> {
if (container instanceof TomcatEmbeddedServletContainerFactory) {
TomcatEmbeddedServletContainerFactory tomcat = (TomcatEmbeddedServletContainerFactory) container;
tomcat.addConnectorCustomizers(
(connector) -> {
connector.setMaxPostSize(10000000); // 10 MB
}
);
}
};
}
Edit: Apparently adding this to your application.properties file will also increase the maxPostSize, but I haven't tried it myself so I can't confirm. multipart.maxFileSize=10Mb # Max file size.
multipart.maxRequestSize=10Mb # Max request size.
| | answeredNov 16 '15 at 0:37 |
|
| | adding the properties works for me: multipart.maxFileSize and multipart.maxRequestSize– Marco WagnerSep 21 '16 at 15:19 | | theconnector solution didn't work for me, neither did themultipart.maxFileSize . What worked for me wasspring.http.multipart.max-file-size from the answer below.– Riki137Sep 9 at 20:54 |
|
| There is some difference when we define the properties in the application.properties and application yaml. In application.yml : spring:
http:
multipart:
max-file-size: 256KB
max-request-size: 256KB
And in application.propeties : spring.http.multipart.max-file-size=128KB spring.http.multipart.max-request-size=128KB Note : Spring version 4.3 and Spring boot 1.4 |
| |
| from documentation:https://spring.io/guides/gs/uploading-files/ Tuning file upload limits When configuring file uploads, it is often useful to set limits on the size of files. Imagine trying to handle a 5GB file upload! With Spring Boot, we can tune its auto-configured MultipartConfigElement with some property settings. Add the following properties to your existingsrc/main/resources/application.properties : spring.http.multipart.max-file-size=128KB
spring.http.multipart.max-request-size=128KB
The multipart settings are constrained as follows: spring.http.multipart.max-file-size is set to 128KB , meaning total file size cannot exceed 128KB.
spring.http.multipart.max-request-size is set to 128KB, meaning total request size for a multipart/form-data cannot exceed 128KB.
|
https://stackoverflow.com/questions/33232849/increase-http-post-maxpostsize-in-spring-boot
其它参考:
http://godjohnny.iteye.com/blog/2352626
http://www.91r.net/ask/33232849.html
这种方式是根据spring boot的EmbeddedServletContainerCustomizer 内嵌tomcat配置实现的
connector.setMaxPostSize(10000000); // 10 MB
,我的程序编译成war包,使用外部tomcat的,所以无法注入
顺便提一下:
spring.http.multipart.max-file-size=100Mb
spring.http.multipart.max-request-size=1000Mb
框架层面(非tomcat层面)在springboot下并没有什么用,springboot中起控制作用的是:
multipart.maxFileSize=100Mb
multipart.maxRequestSize=1000Mb
http://blog.csdn.net/silyvin/article/details/70665377
那么然后自然就想到直接改tomcat的maxpostsize参数,但是动作太大,姑且把这种方法搁置
分析一下情况,tomcat默认2M的maxPostSize,h5 ajax一直可以正常使用,安卓也可以,为什么?
查到:maxPostSize参数只有当request的Content-Type为“application/x-www-form-urlencoded”时起作用。
参考:http://blog.csdn.net/lafengwnagzi/article/details/72846195
As can be seen inRequest.java, maxPostSize is taken into account only for the "application/x-www-form-urlencoded" content type and is not affected by how the request is made ("normal" vs. xhr).
https://stackoverflow.com/questions/7696197/tomcat-maxpostsize-value-ignored-with-xmlhttprequest
于是我找到h5
使用
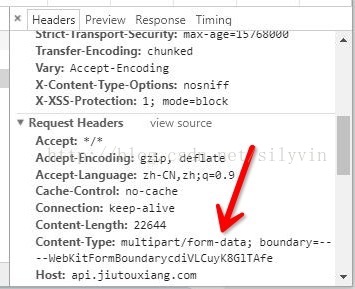
安卓也显式设置 maltipart/form-data,
猜测ios的网络库可能作了些调整
于是找到ios,调试下来发现也是 maltipart/form-data,算了直接修改tomcat 的 maxPostSize吧(默认2M,修改为-1即可,tomcat8之后,0不行了),就这样了
ios使用的网络库为anf